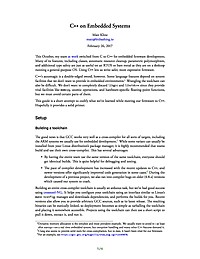
C++ On Embedded Systems
This October, my team at work switched from C to C++ for embedded firmware development. Many of its features, including classes, automatic resource cleanup, parametric polymorphism, and additional type safety are just as useful on an RTOS or bare metal as they are on a desktop running a general-purpose OS. Using C++ lets us write safer, more expressive firmware.
C++'s automagic is a double-edged sword, however. Some language features depend on system facilities that we don't want to provide in embedded environments.* Wrangling the toolchain can also be difficult. We don't want to completely discard libgcc and libstdc++ since they provide vital facilities like memcpy, atomic operations, and hardware-specific floating-point functions, but we must avoid certain parts of them.
This guide is a short attempt to codify what we've learned while moving our firmware to C++. Hopefully it provides a solid primer.

Bare-metal programming for ARM - A hands-on guide
The subject of this ebook is bare-metal programming in C for an ARM system. Specifically, the ARMv7-A architecture is used, which is the last purely 32-bit ARM architecture, unlike the newer ARMv8/AArch64. The -A suix in ARMv7-A indicates the A profile, which is intended for more resource-intensive applications. The corresponding microcontroller architecture is ARMv7-M.
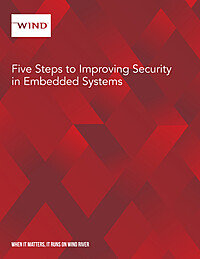
Five Steps to Improving Security in Embedded Systems
In today’s increasingly interconnected world, security breaches are becoming ever more prevalent, with escalating complexity challenges. How can embedded device developers balance the need for tighter security with competing business and market demands? This paper outlines five steps for building additional security assurance into embedded devices by considering the whole product lifecycle.

Interface Protection for HDMI
The High-Definition Multimedia Interface (HDMI) combines a high-speed unidirectional TMDS data link with low speed, bidirectional control and status links (DDC and CEC) and configuration protocols in a single user-friendly highperformance connector.

Creating a State-of-the Art, Cost Effective Energy Harvesting Bluetooth® Low Energy Switch
As IoT rapidly grows into new markets such as MHealth, Agriculture 4.0, and building automation, new questions are being raised about the energy required to support its growth. Within the industry, we see a broad spectrum of power requirements.

Boosting Performance Oscilloscope Versatility, Scalability Whitepaper
Rising data communication rates are driving the need for very high-bandwidth real-time oscilloscopes in the range of 60-70 GHz. These instruments are essential for validating and debugging new designs in coherent optical modulation analysis, high energy physics research, high speed data communications and other areas. With the DPO70000SX Performance Oscilloscope series, Tektronix delivers real-time signal acquisition with an ultra-high bandwidth of 70 GHz, along with a real-time sample rate of 200 GS/s (5ps/sample resolution), making it ideal for such applications.
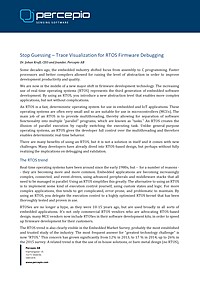
Stop Guessing – Trace Visualization for RTOS Firmware Debugging
Some decades ago, the embedded industry shifted focus from assembly to C programming. Faster processors and better compilers allowed for raising the level of abstraction in order to improve development productivity and quality. We are now in the middle of a new major shift in firmware development technology. The increasing use of real-time operating systems (RTOS) represents the third generation of embedded software development. By using an RTOS, you introduce a new abstraction level that enables more complex applications, but not without complications.
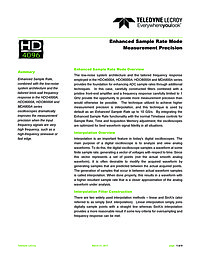
Enhanced Sample Rate Mode Measurement Precision
The low-noise system architecture and the tailored frequency response employed in the HDO4000A, HDO6000A, HDO8000A and MDA800A series provides the foundation for enhancing ADC sample rates through additional techniques. In this case, carefully constructed filters combined with a pristine front-end amplifier and a frequency response carefully limited to 1 GHz provide the opportunity to provide more measurement precision than would otherwise be possible. The technique utilized to achieve higher measurement precision is interpolation, and this technique is used by default as an Enhanced Sample Rate up to 10 GS/s. By integrating the Enhanced Sample Rate functionality with the normal Timebase controls for Sample Rate, Time and Acquisition Memory adjustment, the oscilloscopes are optimized for best waveform signal fidelity in all situations.

Transforming 64-Bit Windows to Deliver Software-Only Real-Time Performance
Next-generation industrial, vision, medical and other systems seek to combine highend graphics and rich user interfaces with hard real-time performance, prioritization and precision.Today’s industrial PCs running 64-bit Windows, complemented by a separate scheduler on multicore multiprocessors, can deliver that precise real-time performance on software-defined peripherals.

Improving Battery Management System Performance and Cost with Altera FPGAs
The purpose of this white paper is to evaluate improvements to Battery Management System (BMS) performance and cost with Altera® FPGAs. In many high-voltage battery systems, including electric vehicles, grid attached storage and industrial applications, the battery is a significant portion of the system cost, and needs to be carefully managed by a BMS to maximize battery life and to optimize charging and discharging performance. This white paper presents the BMS functional requirements for these applications and outlines existing BMS architectures. Key BMS architectural challenges are discussed and opportunities for Altera devices are identified. For each of these opportunities, the performance and cost of the existing solution are compared with Altera FPGA solutions. Altera devices provide architectural flexibility, scalability, customization, performance improvements, and system cost savings in BMS applications.
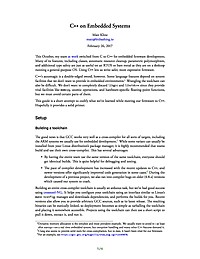
C++ On Embedded Systems
This October, my team at work switched from C to C++ for embedded firmware development. Many of its features, including classes, automatic resource cleanup, parametric polymorphism, and additional type safety are just as useful on an RTOS or bare metal as they are on a desktop running a general-purpose OS. Using C++ lets us write safer, more expressive firmware.
C++'s automagic is a double-edged sword, however. Some language features depend on system facilities that we don't want to provide in embedded environments.* Wrangling the toolchain can also be difficult. We don't want to completely discard libgcc and libstdc++ since they provide vital facilities like memcpy, atomic operations, and hardware-specific floating-point functions, but we must avoid certain parts of them.
This guide is a short attempt to codify what we've learned while moving our firmware to C++. Hopefully it provides a solid primer.

Bare-metal programming for ARM - A hands-on guide
The subject of this ebook is bare-metal programming in C for an ARM system. Specifically, the ARMv7-A architecture is used, which is the last purely 32-bit ARM architecture, unlike the newer ARMv8/AArch64. The -A suix in ARMv7-A indicates the A profile, which is intended for more resource-intensive applications. The corresponding microcontroller architecture is ARMv7-M.
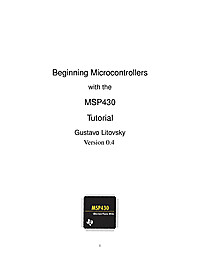
Beginning Microcontrollers with the MSP430 - Tutorial
From the Preface: I decided to write this tutorial after seeing many students struggling with the concepts of programming the MSP430 and being unable to realize their applications and projects. This was not because the MSP430 is hard to program. On the contrary, it adopts many advances in computing that has allowed us to get our application running quicker than ever. However, it is sometimes difficult for students to translate the knowledge they acquired when studying programming for more traditional platforms to embedded systems.

First Steps with Embedded Systems
This book is intended to fill the need for an intermediate level overview of programming microcontrollers using the C programming language. It is aimed specifically at two groups of readers who have different, yet overlapping needs. The first group are familiar with C but require an examination of the general nature of microcontrollers: what they are, how they behave and how best to use the C language to program them. The second group are familiar with microcontrollers but are new to the C programming language and wish to use C for microcontroller development projects. First Steps with Embedded Systems will be useful both as an introduction to microcontroller programming for intermediate level post-secondary programs and as a guide for developers coping with the growth and change of the microcontroller industry.

Memory allocation in C
This article is about dynamic memory allocation in C in the context of embedded programming. It describes the process of dynamically allocating memory with visual aids. The article concludes with a practical data communications switch example which includes a sample code in C.
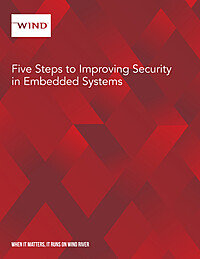
Five Steps to Improving Security in Embedded Systems
In today’s increasingly interconnected world, security breaches are becoming ever more prevalent, with escalating complexity challenges. How can embedded device developers balance the need for tighter security with competing business and market demands? This paper outlines five steps for building additional security assurance into embedded devices by considering the whole product lifecycle.
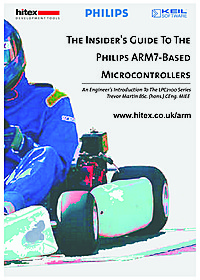
An Engineer's Guide to the LPC2100 Series
This book is intended as a hands-on guide for anyone planning to use the Philips LPC2000 family of microcontrollers in a new design. It is laid out both as a reference book and as a tutorial. It is assumed that you have some experience in programming microcontrollers for embedded systems and are familiar with the C language. The bulk of technical information is spread over the first four chapters, which should be read in order if you are completely new to the LPC2000 and the ARM7 CPU.

Embedded Systems – Theory and Design Methodology
This book addresses a wide spectrum of research topics on embedded systems, including basic researches, theoretical studies, and practical work.

CPU Memory - What Every Programmer Should Know About Memory
As CPU cores become both faster and more numerous, the limiting factor for most programs is now, and will be for some time, memory access. Hardware designers have come up with ever more sophisticated memory handling and acceleration techniques–such as CPU caches–but these cannot work optimally without some help from the programmer. Unfortunately, neither the structure nor the cost of using the memory subsystem of a computer or the caches on CPUs is well understood by most programmers. This paper explains the structure of memory subsystems in use on modern commodity hardware, illustrating why CPU caches were developed, how they work, and what programs should do to achieve optimal performance by utilizing them.
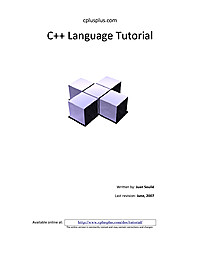
C++ Tutorial
These tutorials explain the C++ language from its basics up to the newest features of ANSI-C++, including basic concepts such as arrays or classes and advanced concepts such as polymorphism or templates. The tutorial is oriented in a practical way, with working example programs in all sections to start practicing each lesson right away